函数需要先定义后调用原则,否则报错
// 定义
function sayHi() {
alert("Hello " + arguments[0] + ", " + arguments[1]);
}
// 或者
var sayHi = function () {
alert("Hello " + arguments[0] + ", " + arguments[1]);
}
sayHi("Nicholas", "how are you today?");
console.log(sayHi.name); //sayHi 输出函数名
arguments 这算是全局参数么?其长度会根据方法调用时传递进来的变化;其是一个数组,索引从0开始
function howManyArgs() {
alert(arguments.length);
}
howManyArgs("string", 45); //2
howManyArgs(); //0
howManyArgs(12); //1
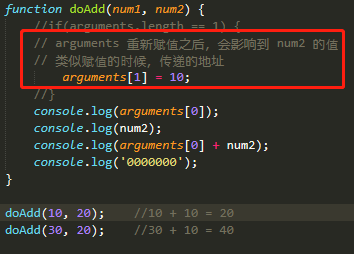
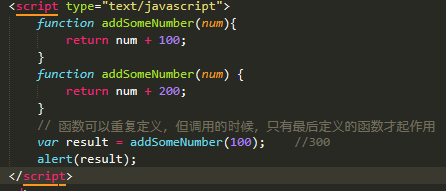
变量作用域
var color = "blue";
function changeColor(){
var anotherColor = "red";
function swapColors(){
var tempColor = anotherColor;
anotherColor = color;
color = tempColor;
//color, anotherColor, and tempColor are all accessible here
}
//color and anotherColor are accessible here, but not tempColor
swapColors();
}
changeColor();
//anotherColor and tempColor aren't accessible here, but color is
alert("Color is now " + color);
function add(num1, num2) {
// var sum = num1 + num2;
// 当使用上面的代码时, alert 处就会产生 error,表示 sum 是不定义的变量
// 但是如下代码时,则可以正确使用
// 提示: 变量声明后再使用,则为局部变量,变量默认的使用域为全局!??
sum = num1 + num2;
return sum;
}
var result = add(10, 20); //30
alert(sum);
function outputNumbers(count){
// 虽然 i 变量是在 for 里面定义的
// 但其作用域却是整个 function
for (var i=0; i < count; i++){
document.write(i);
}
document.write(i); //count
}
outputNumbers(5);
function outputNumbers(count){
for (var i=0; i < count; i++){
document.write(i);
}
document.write("<br/>");
var i; // variable re-declared 虽然重新定义了,但并不会重置其值
document.write(i); //count
}
outputNumbers(5);
function outputNumbers(count){
// 匿名函数,直接调用,在其中定义的变量
// 其作用域也只能在里面访问
(function () {
for (var i=0; i < count; i++){
document.write(i);
}
})();
document.write(i); //causes an error
}
outputNumbers(5);
Function的参数为 object 对象时,是地址传递,函数内对它的操作会影响外面的object
function setName(obj) {
obj.name = "Nicholas";
}
var person = new Object();
setName(person);
console.log(person); //"Nicholas"
函数做为参数传递
function callSomeFunction(someFunction, someArgument){
return someFunction(someArgument);
}
function getGreeting(name){
return "Hello, " + name;
}
var result2 = callSomeFunction(getGreeting, "Nicholas");
console.log(result2); //Hello, Nicholas
对象数组,根据对象指定属性排序的实现
function createComparisonFunction(propertyName) {
return function(object1, object2){
var value1 = object1[propertyName];
var value2 = object2[propertyName];
if (value1 < value2){
return -1;
} else if (value1 > value2){
return 1;
} else {
return 0;
}
};
}
var data = [{name: "Zachary", age: 28}, {name: "Nicholas", age: 29}];
data.sort(createComparisonFunction("name"));
alert(data[0].name); //Nicholas
data.sort(createComparisonFunction("age"));
alert(data[0].name); //Zachary
apply() 、call() 、bind() 方法的应用,其都是用来重定义 this 对象的!参考
// apply()
function sum(num1, num2){
return num1 + num2;
}
function callSum1(num1, num2){
return sum.apply(this, arguments);
}
function callSum2(num1, num2){
return sum.apply(this, [num1, num2]);
}
console.log(callSum1(10,10)); //20
console.log(callSum2(10,10)); //20
// bind()
window.color = "red";
var o = { color: "blue" };
function sayColor(){
console.log(this.color);
}
var objectSayColor = sayColor.bind(o);
objectSayColor(); //blue
// call()
window.color = "red";
var o = { color: "blue" };
function sayColor(){
alert(this.color);
}
sayColor(); //red
sayColor.call(this); //red
sayColor.call(window); //red
sayColor.call(o); //blue
function sum(num1, num2){
return num1 + num2;
}
function callSum(num1, num2){
return sum.call(this, num1, num2);
}
alert(callSum(10,10)); //20
Caller()
function outer(){
inner();
}
// inner.caller 返回调用自己的对象
function inner(){
// console.log(arguments.callee.caller);
console.log(inner.caller);
}
outer();
function factorial(num){
if (num <= 1) {
return 1;
} else {
// 5 * arguments.callee(4)
// 5 * 4 * 3 * 2 * 1
// 有点像递归
return num * arguments.callee(num-1)
}
}
// 函数定义后,可直接赋值给变量
var trueFactorial = factorial;
// 此处相当于重写了 函数,不会影响上面赋值的处理,后续调用会受影响
factorial = function(){
return 0;
};
console.log(trueFactorial(5)); //120
console.log(factorial(5)); //0